Embed React into an HTML web page
Tutorial: Add React to an existing HTML page.
You're in a hurry, you need to quickly add something dynamic to a simple web page. You want to use React because it's a better structure than plain old JavaScript or jQuery. In this tutorial, I'll show you how to create a very simple quiz component using React and embed it into a basic web page.
1. Add React library from the CDN
To keep things simple, we'll link to React, React DOM and Babel from the CDN.
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.4.2/react.js" charset="utf-8"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.4.2/react-dom.js" charset="utf-8"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-standalone/6.21.1/babel.min.js" charset="utf-8"></script>
The three scripts are React (the framework), ReactDOM (to map it to a browser DOM) and Babel (to allow us to use modern JavaScript and compile it for use in the browser in real-time).
2. Create a component
Now create a simple React component, in our case a quiz widget.
<script type="text/babel">
class Quiz extends React.Component {
constructor(props) {
super(props);
this.state = {
correct: null
};
}
answer(correct) {
this.setState({
correct
});
}
render() {
let question = null;
if (this.state.correct === null) {
question = <div>
<button onClick={() => this.answer(false)}>a) A graph database query language</button>
<button onClick={() => this.answer(true)}>b) An API query language</button>
<button onClick={() => this.answer(false)}>c) A graph drawing API</button>
</div>;
}
let answer = null;
if (this.state.correct === true) {
answer = <div className="correct">Correct! It is an API query language</div>;
} else if (this.state.correct === false) {
answer = <div className="incorrect">Nope! It's actually an API query language</div>;
}
return <div className="quiz">
<p>What is GraphQL?</p>
{question}
{answer}
</div>;
}
}
ReactDOM.render(
<Quiz />,
document.getElementById('quiz')
);
</script>
This is a very basic React component which renders a 3 button quiz. When the user clicks on one of the buttons, the component updates telling them if they are correct or not. The quiz renders wherever you place the <div id="quiz"></div>
tag.
You will notice that the embedded script isn't a text/javascript
content type, but a text/babel
content type. This is so we can use modern JavaScript, such as JSX and arrow functions. The babel script we included above will compile this automatically on the page load. Ideally you would precompile the JavaScript, but this of course is a quick and dirty solution.
We can add some styling to the quiz using the <style>
tag.
See it in action
Using the above technique, this quiz has been embedded to this article.
The full code
Here's a full example which you can try out yourself.
<!DOCTYPE html>
<html>
<head>
<title>Developer Quiz</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.4.2/react.js" charset="utf-8"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.4.2/react-dom.js" charset="utf-8"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-standalone/6.21.1/babel.min.js" charset="utf-8"></script>
<style>
.quiz {
display: block;
background: linear-gradient(to bottom, #2a86d6 0%,#7db9e8 100%);
padding: 40px;
color: #ffffff;
}
.quiz button {
display: block;
margin-bottom: 10px;
color: #000000;
}
.quiz .correct {
background-color: #ffffff;
padding: 5px;
color: #11AF08;
font-weight: bold;
}
.quiz .incorrect {
background-color: #ffffff;
padding: 5px;
color: #FF0C0C;
font-weight: bold;
}
</style>
<script type="text/babel">
class Quiz extends React.Component {
constructor(props) {
super(props);
this.state = {
correct: null
};
}
answer(correct) {
this.setState({
correct
});
}
render() {
let question = null;
if (this.state.correct === null) {
question = <div>
<button onClick={() => this.answer(false)}>a) A graph database query language</button>
<button onClick={() => this.answer(true)}>b) An API query language</button>
<button onClick={() => this.answer(false)}>c) A graph drawing API</button>
</div>;
}
let answer = null;
if (this.state.correct === true) {
answer = <div className="correct">Correct! It is an API query language</div>;
} else if (this.state.correct === false) {
answer = <div className="incorrect">Nope! It's actually an API query language</div>;
}
return <div className="quiz">
<p>What is GraphQL?</p>
{question}
{answer}
</div>;
}
}
ReactDOM.render(
<Quiz />,
document.getElementById('quiz')
);
</script>
</head>
<body>
<div id="quiz"></div>
</body>
</html>
Conclusion
There you have it, a very quick way to embed React onto a web page. Should you do this for a production web site, probably not. But it can get you out of bind if you're up against the clock or are working in a code base that doesn't have a proper build process. I'm sure we've all been there 🙂.
If you want to discover a system to keep your notes in a single place and organise them atomically for easier recall, then consider checking out my book Atomic Note-Taking.
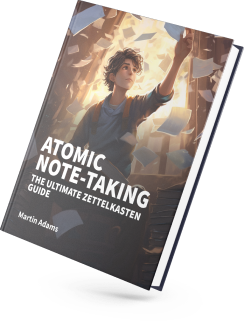